NextJS websites
Tech Stack: NextJS, Typescript, Tailwind, Vercel, Visual Studio Code
The Project
Start Of Development
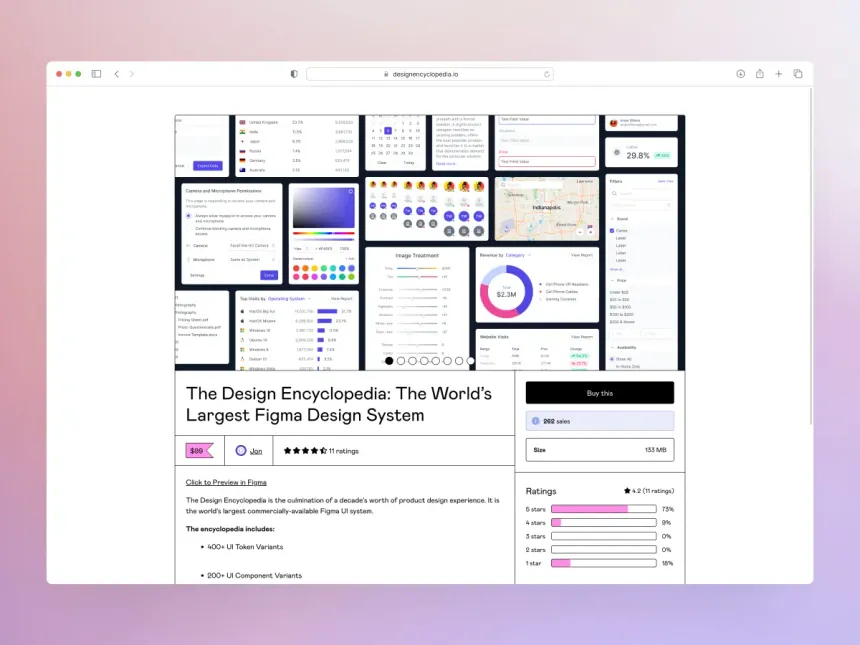
Hosting
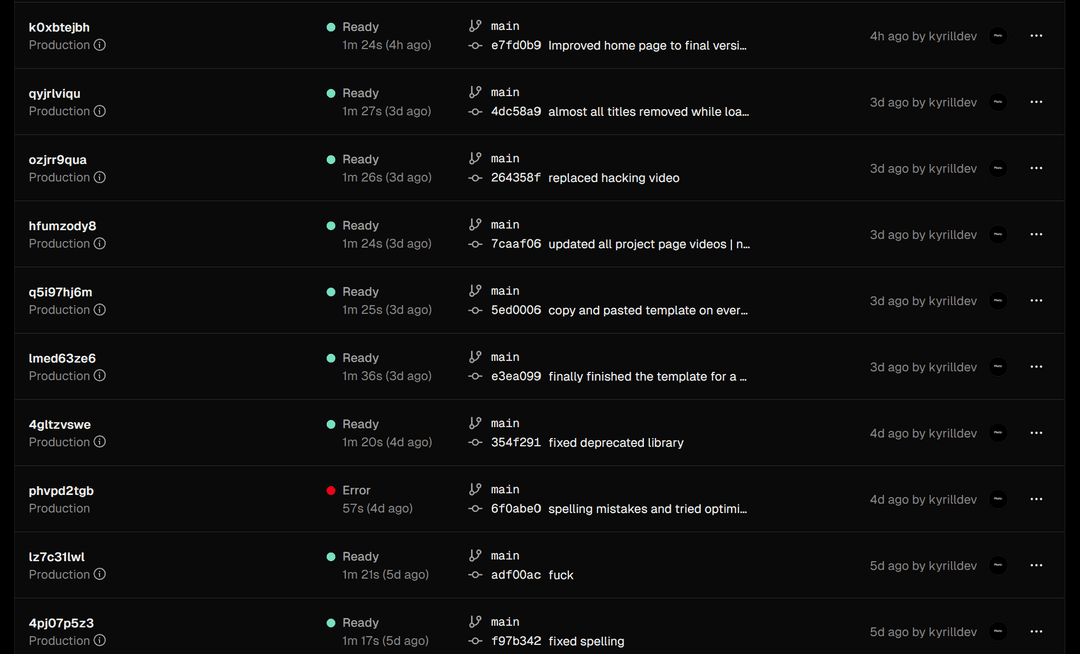
Code Highlights
const isEmailLink = (href: string): boolean => {
return href.startsWith('mailto:');
};
export const SocialLink = ({ className, href, children, icon: Icon }: Props) => {
if (isEmailLink(href))
return (
<li className={clsx(className, 'flex')}>
<Link
href={href}
className="group flex text-sm font-medium text-zinc-800 transition hover:text-primary dark:text-zinc-200">
<Icon className="h-6 w-6 flex-none fill-zinc-500 transition group-hover:fill-primary" />
{children && <span className="ml-4">{children}</span>}
</Link>
</li>);
else
return (
<li className={clsx(className, 'flex')}>
<ExternalLink
href={href}
className="group flex text-sm font-medium text-zinc-800 transition hover:text-primary dark:text-zinc-200">
<Icon className="h-6 w-6 flex-none fill-zinc-500 transition group-hover:fill-primary" />
{children && <span className="ml-4">{children}</span>}
</ExternalLink>
</li>
);
};
Explanation:
This code is part of the Contact box you see on the left bottom corner of this website. I thought I‘d be nice to include my socials everywhere instead of you having to search for them. Each button links to an external website except the email button. Which SHOULD open your email. This code specifically checks if the link provided is a ‘mailto:‘ link or not. In the case that it‘s not you get sent to an external website otherwise you get sent to an internal page.
<div className="absolute w-full overflow-hidden" style={{ paddingTop: '56.25%' }}>
<div className="absolute top-0 left-0 w-full h-full group frame-container">
{project.video && (
<iframe
loading='lazy'
className={clsx(
'absolute top-0 left-0 w-full h-full object-cover opacity-0 transition-opacity duration-500 group-hover:opacity-40 blur-sm',
isHovered && 'opacity-40'
)}
onMouseEnter={() => setIsHovered(true)}
onMouseLeave={() => setIsHovered(false)}
src={project.video}
></iframe>
)}
</div>
</div>
Explanation:
I wrote this code for the background videos on the projects page. I thought it would look much better to make a more interactive projects page instead it just being a static wasteland. So I added a couple project videos in the background, I also blurred the videos for a cleaner look and more readable text on top. I made it so the video never get black bars around it because at the end of the day it is a youtube embed. I‘m not really a fan of the way youtube has implemented their embeds but what are you gonna do...